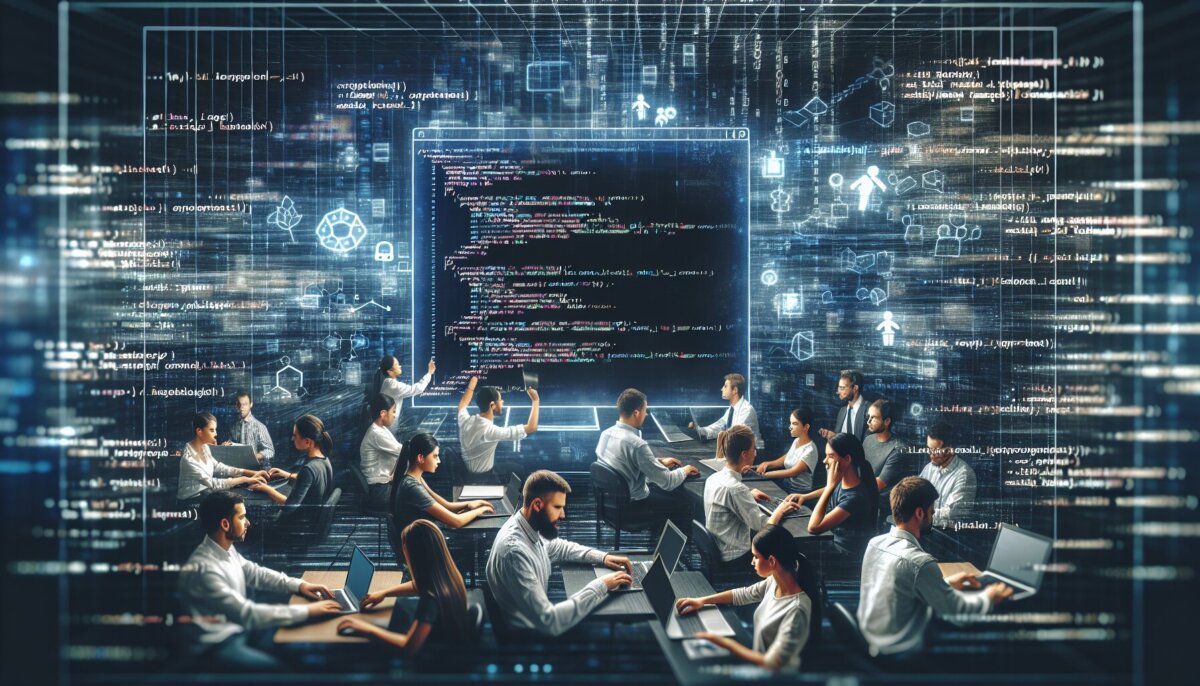
Author: Flavio Pantaleo
· 8 mins read · 478 views
The Power of Object Oriented Programming
Object oriented programming (OOP) stands as a pivotal paradigm in the realm of software development, championing the use of “objects” to encapsulate both data and behavior. This approach, which informs the structure of most modern programming languages, is characterized by its emphasis on classes from which these objects are instantiated, thereby promoting a method of organizing code that enhances flexibility, maintainability, and the reuse of components. OOP not only simplifies the design of complex systems but also aligns with principles such as object-oriented programming polymorphism, contributing to more efficient and adaptable code[1].
By prioritizing methods and design patterns that orient around objects and their interactions, object oriented programming offers a structured solution to tackle the intricacies of software development[2]. This paradigm significantly deviates from procedural programming techniques by focusing on the segmentation of code into discrete, manageable units that embody both functionality and data[1]. Through this lens, OOP concepts like classes and methods serve as the backbone for crafting applications that are not only more robust and scalable but also easier to debug and extend[1][2]. As we delve further into the advantages and real-world applications of OOP, we’ll explore how its inherent properties of flexibility and maintainability set the stage for advancing programming practices and ultimately defining object-oriented programming’s legacy in technology[1][2].
Core Concepts of OOP
1. Encapsulation and Data Hiding
- Encapsulation, a fundamental concept in object oriented programming, involves bundling the data (attributes) and the methods (functions) that manipulate the data into a single unit or class[3][4]. It restricts direct access to some of an object’s components, which can prevent the accidental modification of data[3][4].
- An example of encapsulation in Java is:
publicclassEmployee{private String name;private Date dob;public StringgetName(){return name;}publicvoidsetName(String name){this.name= name;}public DategetDob(){return dob;}publicvoidsetDob(Date dob){this.dob= dob;}}
2. Inheritance for Reusability
- Inheritance allows classes to inherit features from other classes[3][4].
- For example, in Java, one class can inherit from another using the
extends
keyword for class inheritance orimplements
for interfaces. - This mechanism promotes code reusability and can lead to an efficient way of managing code.
3. Polymorphism: Flexibility in Methods
- Polymorphism, meaning “many shapes,” allows methods to do different things based on the object it is acting upon[3][4]. It supports the concept of method overriding and method overloading, enabling actions to vary depending on the object’s class.
- For instance, the
print()
method in Java can handle different types of collections:
publicvoidprint(Collection<String> collection){for(String s: collection){System.out.println("s = "+ s);}}
4. Abstraction: Simplifying Complexity
- Abstraction simplifies complexity by allowing programmers to focus on the interface instead of the specific implementation[3][4]. It hides the detailed workings of a class, only exposing operations relevant for the other objects to interact with. This concept enhances modularity and helps in managing complex systems.
These core principles —Encapsulation, Inheritance, Polymorphism, and Abstraction— are crucial for developing robust, scalable, and maintainable software in various object-oriented programming languages like Java, C++, and Python[3][4][5].
Advantages of Object Oriented Programming
1. Modularity and Troubleshooting
- Object Oriented Programming (OOP) enhances modularity, allowing developers to isolate and address issues within specific objects or classes without impacting others. This encapsulation ensures that objects are self-contained, simplifying both development and problem-solving processes[6].
2. Code Reusability and Efficiency
- Through inheritance, OOP promotes code reusability by enabling new objects to inherit properties from existing ones. This not only saves time but also reduces errors by minimizing code duplication. Subclasses can extend generic classes, adding unique attributes while leveraging already tested and verified code, thereby enhancing efficiency and reliability.
3. Security and Data Protection
- OOP provides robust security features by supporting data hiding. This prevents external functions from accessing or modifying the internal state of the object, thus safeguarding data integrity and minimizing the risk of data corruption[5].
4. Flexibility and Scalability
- Polymorphism in OOP allows methods to use objects of different classes, enabling a single function to adapt to different classes. This flexibility makes it easier to scale applications and modify them with minimal disruptions. Additionally, OOP’s focus on objects rather than procedures aids in managing larger projects and adapting to new problem-solving scenarios effectively.
5. Enhanced Productivity and Maintenance
- OOP frameworks facilitate quicker program development due to the availability of multiple libraries and reusable codes. This significantly boosts productivity. Moreover, the clear modular structure and encapsulation make maintenance and upgrades easier and less costly[6].
6. Simplified Complex Systems Handling
- OOP excels in managing real-time complexities, providing tools to adapt, modify, and reuse code efficiently. This is particularly advantageous in large, complex system developments where traditional procedural approaches might falter.
7. Interface Simplicity
- The use of message passing techniques for object communication simplifies the descriptions of external systems, making interfaces cleaner and more straightforward to manage. This abstraction helps in reducing system complexity and enhancing interaction between different software components[6].
Comparison with Other Programming Paradigms
Object Oriented Programming (OOP) and procedural programming represent two fundamentally different approaches to coding, each with unique characteristics and advantages. OOP, unlike procedural programming, encapsulates data and behavior into objects, an approach that mirrors real-world interactions more closely than the abstract procedures used in traditional programming models[5].
Key Differences:
1. Structural Approach:
- OOP divides a program into objects, integrating state and behavior, which contrasts sharply with procedural programming that organizes code into functions and separates data and procedures[5].
2. Problem-Solving Orientation:
- OOP employs a bottom-up approach in problem-solving, starting with specifics and integrating them into complex structures. Conversely, procedural programming takes a top-down approach, breaking down the programming tasks into smaller sub-tasks[5].
3. Real vs. Unreal World Modeling:
- OOP is modeled after the real world, making it intuitive for managing real-world complexities. Procedural programming, on the other hand, is considered to be based on an ‘unreal’ world, focusing more on the process than on data.
4. Scale of Application:
- OOP is typically used for larger, more complex applications due to its scalability and robustness, whereas procedural programming is suited for medium-sized projects.
5. Abstraction Level:
- The level of abstraction in OOP is centered around data abstraction, which is different from procedural programming that focuses on procedural abstraction.
Programming Models Comparison:
Procedural Programming Languages:
- Include FORTRAN, ALGOL, COBOL, BASIC, Pascal, and C[5].
Object-Oriented Programming Languages:
- Widely used languages include Java, C++, and Python, which support the creation and manipulation of objects[5].
In recent years, functional programming (FP) has also gained traction, distinguished by its focus on transforming objects rather than facilitating interactions between them, presenting yet another paradigm shift in programming methodologies. This approach emphasizes immutability and stateless functions, which can lead to more predictable and bug-resistant code.
Real-World Applications of OOP
Object oriented programming (OOP) is integral to numerous sectors, demonstrating its versatility and effectiveness in handling complex and varied applications.
Below are key real-world applications of OOP, showcasing its broad utility across different industries and systems:
1. Banking and Financial Systems
- Scalable Architectures: OOP facilitates the development of scalable architectures in banking systems, enabling efficient management of financial transactions and customer data.
- Secure Transactions: Enhanced security features inherent in OOP help in safeguarding sensitive financial information.
2. Educational Systems
- School Management Systems: OOP principles are applied to create modular and scalable school management systems, improving administrative efficiency and educational service delivery.
- Client-Server Systems: Object-oriented client-server systems in education enhance IT infrastructure, supporting robust digital learning platforms.
3. Healthcare Systems
- Medical Expert Systems: OOP aids in developing expert systems that analyze problems and diagnose conditions, significantly improving decision-making in healthcare.
- Real-Time Monitoring: Real-time systems designed using OOP techniques manage patient monitoring with strict timing constraints, ensuring timely medical interventions.
4. E-Commerce
- Shopping Platforms: OOP enhances the scalability and efficiency of e-commerce systems, making online shopping platforms more robust and user-friendly.
- Product and Cart Management: Python classes are used to model products and shopping carts, facilitating features like item additions and total price calculations.
5. Engineering Applications
- CAD/CAM Systems: In computer-aided design and manufacturing, OOP simplifies the creation of detailed blueprints and flowcharts, enhancing precision and efficiency.
- Simulation Software: OOP is used in developing simulation software that models real-world physics and engineering scenarios, aiding in complex system analyses.
6. Entertainment and Media
- Video Game Development: The gaming industry heavily relies on OOP for developing interactive and high-performance video games that offer dynamic user experiences.
- Media Management: Object-oriented databases like MongoDB maintain a direct correspondence between media content objects and database objects, optimizing data integrity and retrieval.
7. Scientific Research
- Ecological Simulations: OOP is employed to model ecological systems, simulating interactions within ecosystems to study environmental impacts and conservation strategies.
- Neural Networks: In research, OOP simplifies the development of neural networks used in predictive modeling and data analysis, particularly in parallel computing environments.
These applications illustrate the profound impact of OOP in streamlining operations, enhancing system functionality, and providing tailored solutions across diverse fields.
Challenges and Criticisms of OOP
1. Understanding the Limitations of OOP
- Object oriented programming (OOP) is widely recognized for its ability to organize complex code through the use of objects, classes, and methods.
However, it also faces significant challenges and criticisms that affect its effectiveness in certain scenarios[7]:
2. Complexity in Object Management:
- As the scale of a project increases, managing objects can become cumbersome. Developers often find that objects become ‘god objects’—overly generic and difficult to maintain. This complexity can deter the scalability and maintainability that OOP aims to provide.
3. Mutable State Issues:
- Mutable objects in OOP can lead to unexpected behavior across different parts of the code, especially when the object’s state is changed. This issue is exacerbated by Object-Relational Mapping (ORM) systems that are typically mutable, leading to potential conflicts when multiple parts of an application interact with the same database objects.
4. Educational Challenges:
- The abstract concepts of OOP such as classes, instances, and the purpose of objects can be difficult for new developers to grasp. This steep learning curve can hinder the adoption of OOP principles effectively.
5. Performance Overheads:
- Implementing OOP features like inheritance, encapsulation, and polymorphism can introduce unnecessary overheads, potentially impacting the performance of applications, especially in systems where efficiency is critical.
6. Tight Coupling Between Classes:
- OOP can lead to tightly coupled classes, which makes the system more rigid and less adaptable to changes. This coupling can complicate modifications and extensions of the system.
7. Inflexibility in Dynamic Environments:
- The class-based structure of OOP may not always be suitable in environments that require dynamic behavior or runtime changes in the object structure.
8. Memory Management Challenges:
- In languages that do not automatically manage memory, OOP can lead to issues such as memory leaks, which occur when memory that is no longer needed is not released properly.
9. Real-World Representation:
- OOP often simplifies real-world entities into classes and objects, which might not always accurately represent the complexities or the dynamic nature of real-world systems.
- For example, representing the animal kingdom in a class hierarchy can be overly simplistic and not reflective of its true diversity.
10. Deep Hierarchies:
- Deep class hierarchies in OOP can make the code difficult to understand and test, leading to less maintainable systems.
11. Myths of Reusability:
- While OOP promotes reusability, in large systems, this often leads to code bloat and increased complexity rather than streamlined and efficient code reuse.
These challenges highlight the importance of understanding both the strengths and limitations of OOP. By acknowledging these criticisms, developers can better decide when and how to implement OOP effectively in their projects.
Conclusion
Throughout this exploration of Object Oriented Programming (OOP), we’ve delved into the foundational principles that make it a distinguished paradigm in software development. This discussion has illuminated how encapsulation, inheritance, polymorphism, and abstraction collectively contribute to creating robust, scalable, and maintainable applications across diverse fields. By emphasizing the modular and reusable nature of code, OOP not only enhances the development process but also fortifies the adaptability and efficiency of systems in real-world applications. These characteristics underscore the profound impact OOP has had on programming practices, paving the way for more sophisticated and flexible software solutions.
However, it’s also crucial to acknowledge the challenges and criticisms associated with OOP, which stem from issues like object management complexity, mutable state concerns, and the steep learning curve for new developers. Recognizing these limitations allows for a more nuanced application of OOP principles, ensuring that developers can leverage its strengths while mitigating potential drawbacks. As we move forward, the continuous evolution of OOP and its integration with other programming paradigms promise to further broaden its utility and effectiveness in addressing the complexities of modern software development. Embracing both the advantages and challenges of OOP enables the creation of innovative solutions that are tailored to meet the ever-changing demands of technology.
Looking for Expert IT Solutions?
Subscribe to Our Newsletter for Exclusive Tips and Updates!
Stay ahead of tech challenges with expert insights delivered straight to your inbox. From solving network issues to enhancing cybersecurity and streamlining software integration, our newsletter offers practical advice and the latest IT trends. Sign up today and let us help you make technology work seamlessly for your business!
FAQs
What makes object oriented programming so powerful?
Object oriented programming (OOP) is a powerful model because it organizes data into classes and objects, each with their own attributes. This approach is widely used and is a crucial skill for effective communication with programmers within an organization.
How does object oriented programming offer flexibility?
Flexibility in object oriented programming refers to the capacity to adjust to new requirements and enhance functionality without disrupting the existing codebase. It is a balancing act between being adaptable and ensuring the code remains maintainable, which includes being easy to understand, test, debug, and modify.
In what ways is object oriented programming considered more efficient?
Object oriented programming is deemed more efficient because it reduces both the file size and the amount of programming work required. By utilizing flexible objects that already contain necessary methods, there is no need to duplicate code in different parts of the codebase. Moreover, these self-contained objects can be reused in other projects, accelerating development.
What is the primary advantage of using object oriented programming?
The principal benefit of object oriented programming is its ability to break down a complex software system into smaller, manageable objects, with each object handling a distinct function. This modularity allows developers to create, test, and maintain these units independently, streamlining the development process.
Looking for Expert IT Solutions?
Subscribe to Our Newsletter for Exclusive Tips and Updates!
Stay ahead of tech challenges with expert insights delivered straight to your inbox. From solving network issues to enhancing cybersecurity and streamlining software integration, our newsletter offers practical advice and the latest IT trends. Sign up today and let us help you make technology work seamlessly for your business!
References
[1] –https://www.geeksforgeeks.org/introduction-of-object-oriented-programming/
[2] –https://www.youtube.com/watch?v=SiBw7os-_zI
[3] –https://www.interviewbit.com/blog/principles-of-oops/
[4] –https://www.masaischool.com/blog/introduction-to-object-oriented-programming/
[5] –https://www.geeksforgeeks.org/differences-between-procedural-and-object-oriented-programming/
[6] –https://softwareengineering.stackexchange.com/questions/230604/what-exactly-is-procedural-programming-how-exactly-is-it-different-from-oop-is
[7] –http://www.skillsire.com/read-blog/345_analysis-of-different-issues-faced-in-object-oriented-programming.html
Share